Log important JavaScript events
Insert loggers in your JavaScript
JL().info("log message");
Optionally use logger names
JL("jsLogger").info("log message");
Log JavaScript exceptions, with their stack traces
try { ... } catch(e) { JL().fatalException("Exception info", e); }
Configure loggers in your JavaScript code
JL("jsLogger").setOptions({ "level": 4000 });
Only log for certain user agents or IP addresses
JL().setOptions( { "userAgentRegex": "MSIE 7|MSIE 8" });
Log objects as well as strings
var obj = {"f1": "v1", "f2": "v2"}; JL().log(2500, obj);
Pass in function that returns log info
JL().log(2500, function() { // Only executed when log info will actually be logged. var loginfo = expensiveOperation(); return loginfo; });
See which messages belong to the same request
Request ID | Message |
---|---|
CB0734CE.... | A debug message |
D623A22B.... | An error message |
CB0734CE.... | Some other message |
D623A22B.... | Yet another message |
D623A22B.... | A fatal message |
... |
Buffers messages whilst off line
Filter out what you don't need
Only log high severity messages
// Only log messages with severity ERROR or higher
JL().setOptions({ "level": JL.getErrorLevel() });
Suppress messages that match a regular expression
JL("jsLogger").setOptions( { "disallow": "suppress me" });
Limit total messages sent to server
JL.setOptions({"maxMessages": 3});
Reduce AJAX requests by batching log messages
appender.setOptions( { "batchSize": 3, "batchTimeout": 1000 });
Easily send logging data to the server
Suppress duplicate messages
Logger | Message |
---|---|
jsLogger | x too high (x = 5) |
jsLogger | x too high (x = 6) |
jsLogger | x too high (x = 7) |
jsLogger | x too high (x = 8) |
jsLogger | x too high (x = 9) |
Get the info you need, but only when you need it
- To solve JavaScript exceptions, you often need to know what happened before they happened.
- But you don't want to send trace messages with that context unless there actually is an exception.
- Solution: JSNLog lets you buffer log messages on the client, and only send them when there is a fatal message.
Show log data in the console
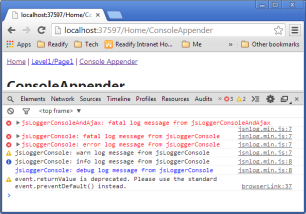