Install JSNLog for .Net Core
version 3.0.3
Automatically logs JavaScript exceptions to your server side logs. Lets you log other client side events such as AJAX time outs. Buffers log messages when user's device loses Internet connection.
1. Install JSNLog
cd <web project directory> dotnet add package JSNLog
2. Add to Startup class
using JSNLog; public void Configure(..., ILoggerFactory loggerFactory) { ... // Add before UseStaticFiles app.UseJSNLog(loggerFactory); ...
3. Done
Uncaught JavaScript exceptions will now get logged in your server side logs.
Why use JSNLog
- You probably log server side exceptions and other events using a logging package such as Serilog, Log4Net, or NLog.
- However, you're not logging JavaScript events. This makes fixing bugs in live JavaScript code much harder.
- Fix this by installing JSNLog. It automatically logs client side exceptions to your server side log.
-
Log other JavaScript events as well, such as AJAX timeouts:
JL().error("..message..");
- JSNLog sends the log data to the server and into your server side log.
- Your logs now have both the server and client side events. You can correlate them with request ids.
- Simple well documented demo projects
- Install JSNLog
6 minute introduction
Vital Stats
- Supports IE 8+, Firefox, Chrome, Opera, Safari (desktop/mobile).
- Free, open source (MIT).
- Use stand alone, as an AMD module, ES6 module or CommonJS module with Browserify, or with bundles. Available on cdnjs.
- Use as an ASP.NET Core Middleware Component with .NETFramework 4.5.2 or .NET Core 2 and higher
- No dependencies on other JavaScript libraries.
- Fully documented: API docs, examples, how to's, videos.
Log important JavaScript events
Insert loggers in your JavaScript
JL().info("log message");
Optionally use logger names
JL("jsLogger").info("log message");
Log JavaScript exceptions, with their stack traces
try { ... } catch(e) { JL().fatalException("Exception info", e); }
Or configure them in your JavaScript code
JL("jsLogger").setOptions({ "level": 4000 });
Log objects as well as strings
var obj = {"f1": "v1", "f2": "v2"}; JL().log(2500, obj);
Pass in function that returns log info
JL().log(2500, function() { // Only executed when log info will actually be logged. var loginfo = expensiveOperation(); return loginfo; });
See which messages belong to the same request
Request ID | Message |
---|---|
CB0734CE.... | A debug message |
D623A22B.... | An error message |
CB0734CE.... | Some other message |
D623A22B.... | Yet another message |
D623A22B.... | A fatal message |
... |
Buffers messages whilst off line
Use a single logging end point for multiple sites
<jsnlog defaultAjaxUrl="http://my-api-domain.com/jsnlog.logger" corsAllowedOriginsRegex="^http://my-xyz-domain[.]com$"> </jsnlog>
Filter out what you don't need
Only log high severity messages
// Only log messages with severity ERROR or higher
<logger level="ERROR" />
Suppress messages that match a regular expression
<logger disallow="suppress me" />
Only log for certain user agents or IP addresses
<logger userAgentRegex="MSIE 7|MSIE 8" />
Limit total messages sent to server
<jsnlog maxMessages="3">
Reduce AJAX requests by batching log messages
<ajaxAppender name="appender1" batchSize="3" batchTimeout="1000"/>
Suppress duplicate messages
Logger | Message |
---|---|
jsLogger | x too high (x = 5) |
jsLogger | x too high (x = 6) |
jsLogger | x too high (x = 7) |
jsLogger | x too high (x = 8) |
jsLogger | x too high (x = 9) |
Get the info you need, but only when you need it
- To solve JavaScript exceptions, you often need to know what happened before they happened.
- But you don't want to send trace messages with that context unless there actually is an exception.
- Solution: JSNLog lets you buffer log messages on the client, and only send them when there is a fatal message.
Show log data in the console
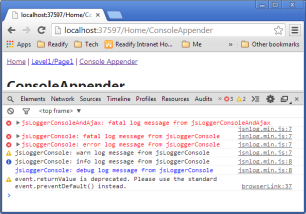